SQL Dialects – Understanding the Variations Across Platforms
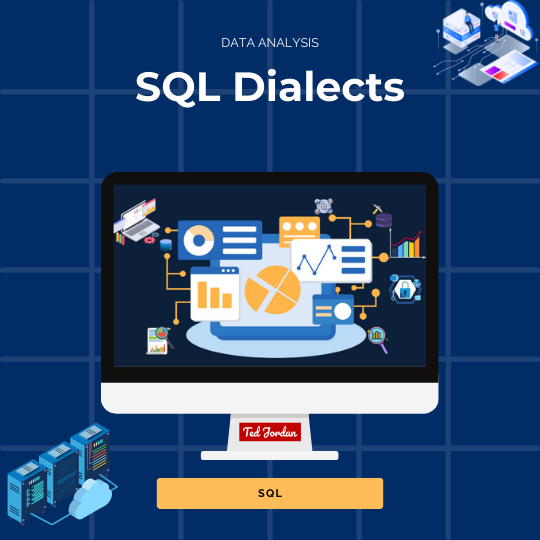
Discover the differences between popular SQL dialects like MySQL, PostgreSQL, SQL Server, Oracle, and SQLite, and learn how to adapt your queries for different environments. SQL Dialect Variations Standard SQL MySQL Auto Increment: AUTO_INCREMENT Limit Syntax: LIMIT 10 PostgreSQL Auto Increment: SERIAL Limit Syntax: LIMIT 10 SQL Server Auto Increment: IDENTITY(1,1) Limit Syntax: TOP 10 […]
SQL Best Practices – Writing Clean, Secure, and Efficient Queries
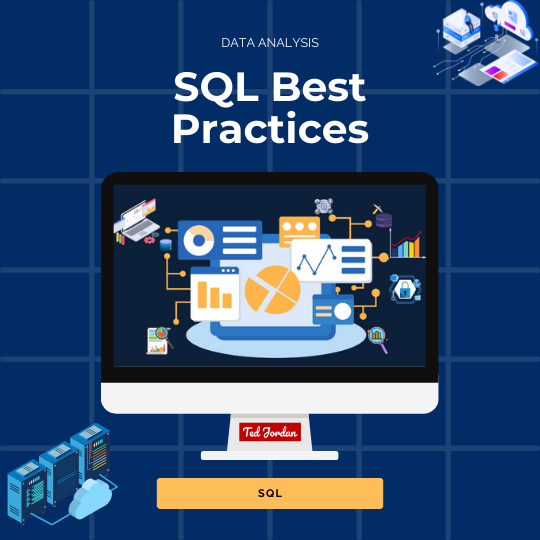
Learn essential SQL best practices to write queries that are not only clean and secure but also optimized for performance. SQL Best Practices Quality SQL Clean & Readable • Consistent formatting • Clear naming conventions Secure • Parameterized queries • Proper permissions Efficient • Proper indexing • Avoid SELECT * Maintainable • Comprehensive comments • […]
Troubleshooting Common SQL Errors – Debugging Tips and Tricks
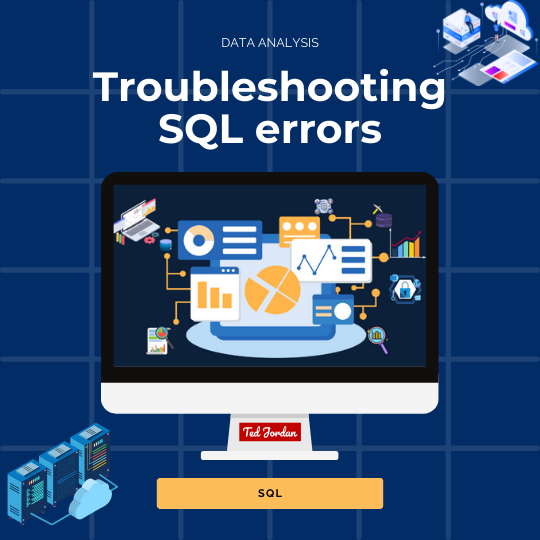
Learn how to diagnose and fix common SQL errors with practical debugging strategies and tips. Introduction Even seasoned SQL developers encounter errors in their queries. Whether it’s a syntax mistake, a missing table, or a logic error, understanding how to troubleshoot and debug SQL queries is an essential skill. In this article, you’ll learn: For […]
Working with Stored Procedures, Functions, and Triggers in SQL
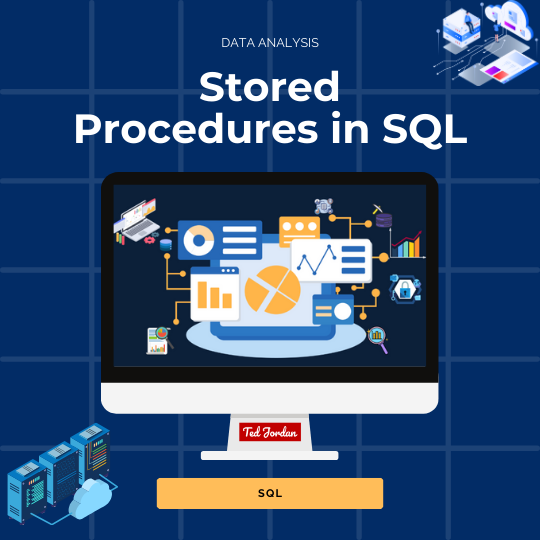
Learn how to automate and encapsulate your SQL code with stored procedures, functions, and triggers for more efficient database operations. SQL Database Objects Stored Procedure Performs an action Returns 0+ result sets CALL GetCustomers() CREATE PROCEDURE GetCustomers() BEGIN SELECT * FROM customers WHERE active = 1; END Function Returns a single value Used in queries […]
Understanding SQL Transactions – COMMIT, ROLLBACK, and ACID Properties
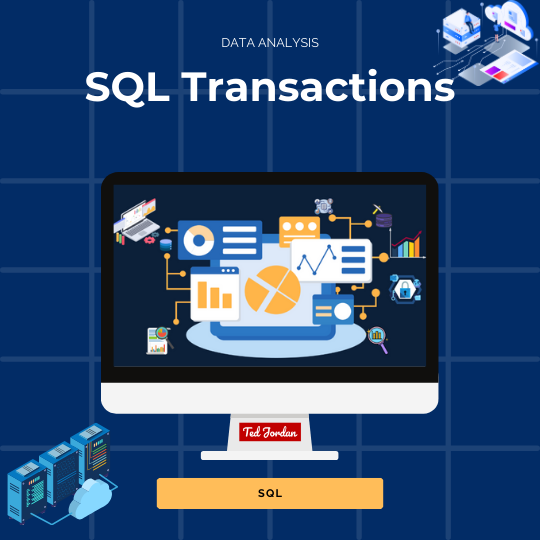
Learn how SQL transactions ensure data integrity through COMMIT, ROLLBACK, and the ACID properties, and discover best practices for managing transactional operations. SQL Transaction Flow BEGIN Database Operations COMMIT Changes Saved ROLLBACK Changes Undone Alt text: Diagram illustrating the concepts of SQL transactions, including COMMIT, ROLLBACK, and ACID properties Introduction As you advance in SQL, […]
Optimizing SQL Queries – Tips for Better Performance
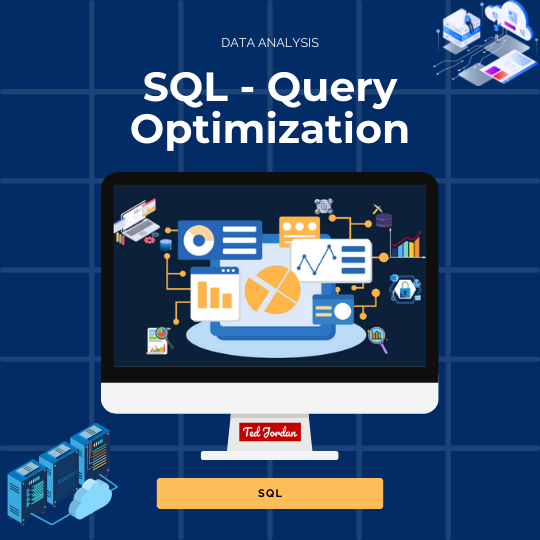
Learn best practices and strategies for optimizing your SQL queries to achieve faster response times and improved performance. SQL Query Optimization Slow Query Optimized Query SELECT * FROM users WHERE LOWER(email) LIKE ‘%gmail%’ ORDER BY created_at; SELECT id, name, email FROM users WHERE email LIKE ‘%gmail%’ 1200ms 120ms 10x faster Key Improvements • Select only […]
Defining Your Database – SQL Data Definition Language (DDL) Basics
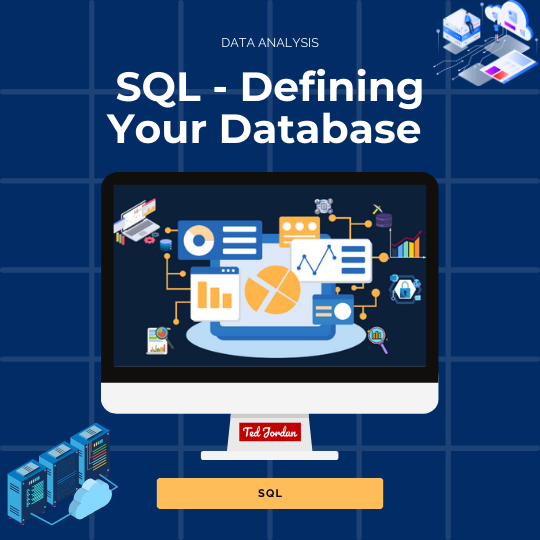
Learn how to create, modify, and manage your database structures using SQL’s Data Definition Language (DDL). SQL Data Definition Language (DDL) Components CREATE TABLE Define new tables INDEX Improve query performance VIEW Virtual tables CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(100), department VARCHAR(50), salary DECIMAL(10,2) ); ❌ No Table ➜ id name […]
Data Manipulation in SQL – INSERT, UPDATE, and DELETE Essentials
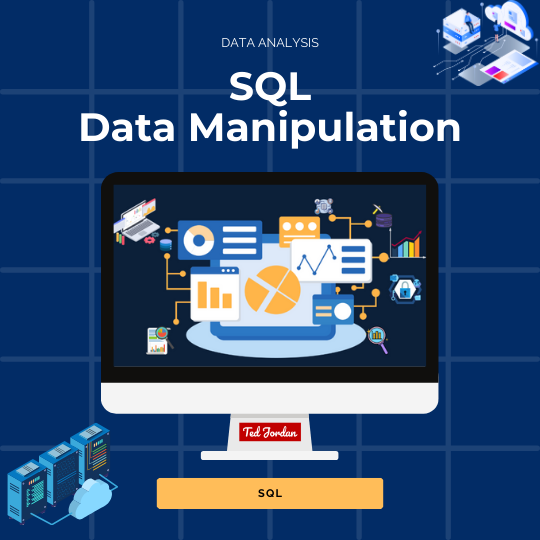
Learn how to modify your data using SQL’s core Data Manipulation Language (DML) commands: INSERT, UPDATE, and DELETE. SQL Data Manipulation Operations INSERT INSERT INTO employees (id, name, department, salary) VALUES (4, ‘Alice’, ‘Marketing’, 65000); id name department salary 1 John IT 75000 2 Sarah HR 65000 3 Mike Sales 70000 ➜ id name department […]
Demystifying SQL Functions – Aggregate, Scalar, and Window Functions
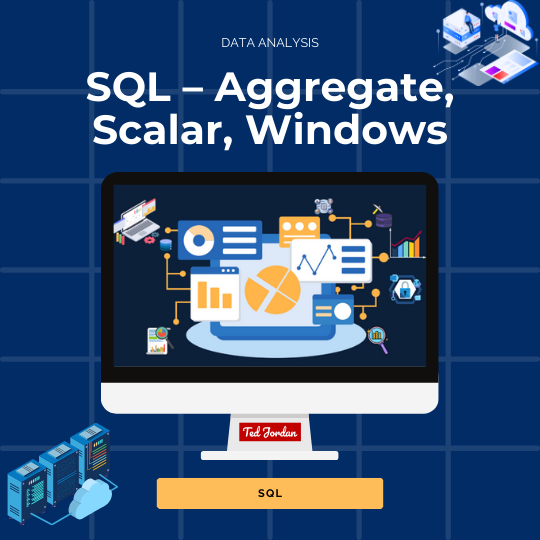
Unlock the power of SQL by mastering its functions. Learn how aggregate, scalar, and window functions can transform your data analysis. SQL Functions Comparison Aggregate Functions Example: SUM, COUNT, AVG – Combines multiple rows – Returns single value – Used with GROUP BY Scalar Functions Example: UPPER, LOWER, LENGTH – Operates row by row – […]
Using Subqueries in SQL – A Guide to Nested Queries
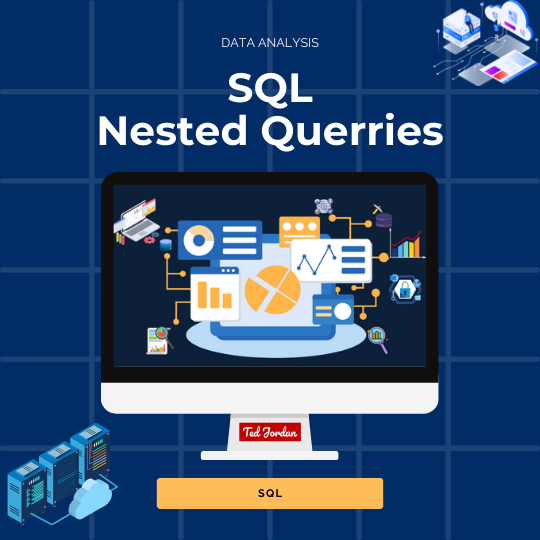
Learn how to leverage subqueries to perform complex data retrieval in SQL by embedding one query within another. Nested Query Structure SELECT customer_name, total_orders FROM customers WHERE total_orders > ( SELECT AVG(total_orders) FROM customers WHERE region = ‘North’ ); Outer Query Inner Query (Subquery) Executes First Returns Single Value How it works: Inner query executes […]